2023. 12. 28. 14:00ㆍ카테고리 없음
Project Manager 에서 Input System 을 다운받아 준 뒤,
Input Action을 만들어준다.
Move - Value Vector2
Look - Value Vector2
Attack - Button
Jump - Button
Interact - Button
다양한 인풋 액션을 만들어줌으로써 스크립트에 일일히 작성하지 않아도 되는 장점이 있다.
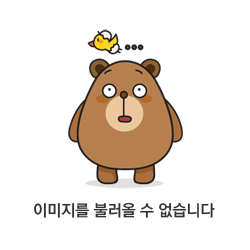
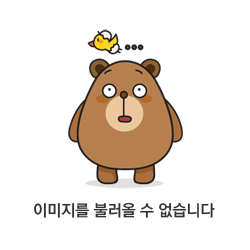
플레이어 오브젝트에 Player Input 을 넣어주고 Player Controls 인풋 액션을 넣어준다.
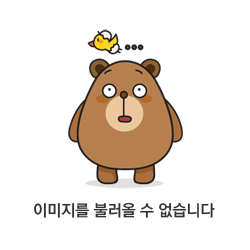
동작들을 수행할 수 있도록 Player Controller 스크립트를 만들어주고 똑같이 Player 오브젝트에 넣어준다.
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEditor.Build;
using UnityEngine;
using UnityEngine.InputSystem;
public class PlayerController : MonoBehaviour
{
[Header("Movement")] //움직임에 관련된 코드
public float moveSpeed;
private Vector2 curMovementInput;
public float jumpForce;
public LayerMask groundLayerMask;
[Header("Look")] //시야에 관련된 코드
public Transform cameraContainer;
public float minXLook;
public float maxXLook;
private float camCurXRot;
public float lookSensitivity;
private Vector2 mouseDelta; //마우스의 움직임에 따라 카메라 시선이 이동한다.
[HideInInspector]
public bool canLook = true;
private Rigidbody _rigidbody;
public static PlayerController instance;
private void Awake()
{
instance = this;
_rigidbody = GetComponent<Rigidbody>();
}
void Start()
{
Cursor.lockState = CursorLockMode.Locked;
}
private void FixedUpdate() //물리적인 처리를 위해 사용
{
Move();
}
private void LateUpdate()
{
if(canLook)
{
CameraLook();
}
}
private void Move()
{
Vector3 dir = transform.forward * curMovementInput.y + transform.right * curMovementInput.x;
dir *= moveSpeed;
dir.y = _rigidbody.velocity.y;
_rigidbody.velocity = dir;
}
void CameraLook()
{
camCurXRot += mouseDelta.y * lookSensitivity;
camCurXRot = Mathf.Clamp(camCurXRot,minXLook,maxXLook);
cameraContainer.localEulerAngles = new Vector3(-camCurXRot, 0, 0);
transform.eulerAngles += new Vector3(0, mouseDelta.x * lookSensitivity, 0);
}
public void OnLookInput(InputAction.CallbackContext context)
{
mouseDelta = context.ReadValue<Vector2>();
}
public void OnMoveInput(InputAction.CallbackContext context)
{
if(context.phase == InputActionPhase.Performed)
{
curMovementInput = context.ReadValue<Vector2>();
}
else if(context.phase == InputActionPhase.Canceled)
{
curMovementInput = Vector2.zero;
}
}
public void OnJumpInput(InputAction.CallbackContext context)
{
if(context.phase == InputActionPhase.Started)
{
if(IsGrounded())
_rigidbody.AddForce(Vector2.up * jumpForce, ForceMode.Impulse);
}
}
private bool IsGrounded()
{
Ray[] rays = new Ray[4]
{
new Ray(transform.position + (transform.forward * 0.2f) + (Vector3.up * 0.01f) , Vector3.down),
new Ray(transform.position + (-transform.forward * 0.2f)+ (Vector3.up * 0.01f), Vector3.down),
new Ray(transform.position + (transform.right * 0.2f) + (Vector3.up * 0.01f), Vector3.down),
new Ray(transform.position + (-transform.right * 0.2f) + (Vector3.up * 0.01f), Vector3.down),
};
for(int i = 0; i < rays.Length; i++)
{
if (Physics.Raycast(rays[i], 0.1f, groundLayerMask))
{
return true;
}
}
return false;
}
private void OnDrawGizmos()
{
Gizmos.color = Color.red;
Gizmos.DrawRay(transform.position + (transform.forward * 0.2f), Vector3.down);
Gizmos.DrawRay(transform.position + (-transform.forward * 0.2f), Vector3.down);
Gizmos.DrawRay(transform.position + (transform.right * 0.2f), Vector3.down);
Gizmos.DrawRay(transform.position + (-transform.right * 0.2f), Vector3.down);
}
public void ToggleCursor(bool toggle)
{
Cursor.lockState = toggle ? CursorLockMode.None : CursorLockMode.Locked;
canLook = !toggle;
}
}
스크립트 작성 된 코드에 대한 이해가 전혀 되지 않아서 공부가 필요할 것 같다.